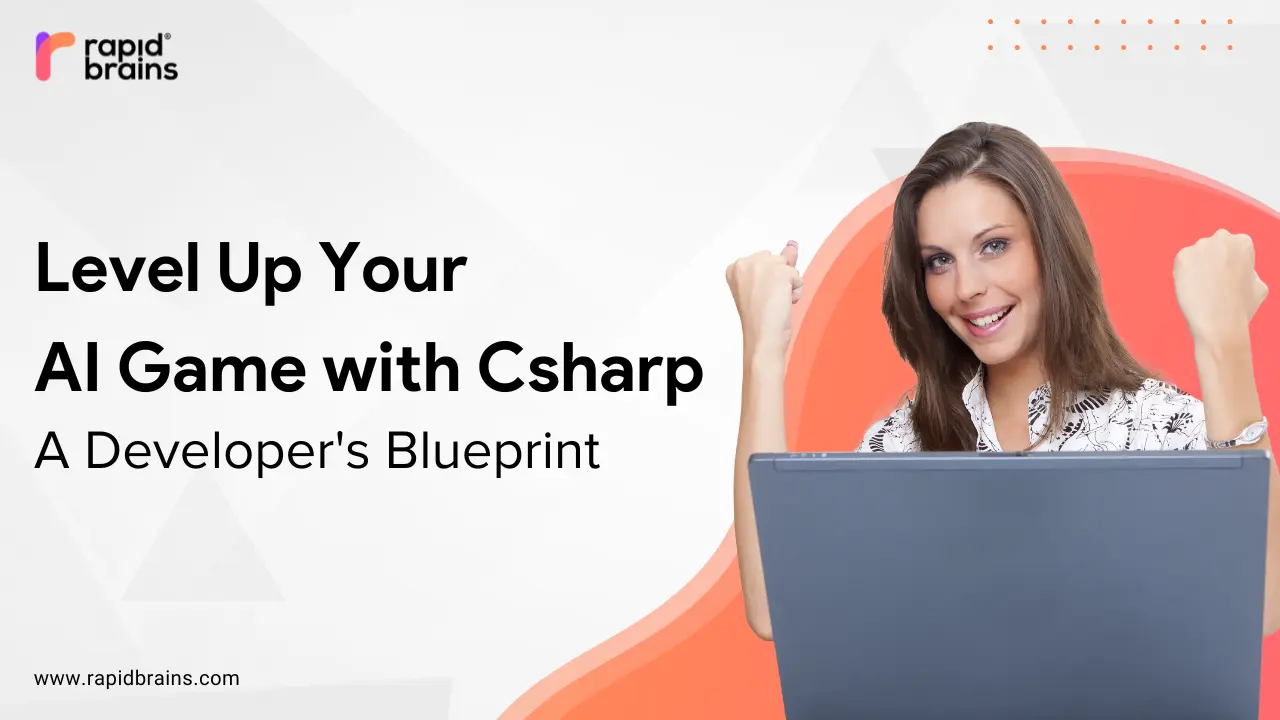
Introduction
Artificial intelligence (AI) is transforming industries by enabling machines to perform tasks that once required human intelligence. C# has become a powerful language for AI development due to its robust features, performance, and vast ecosystem of libraries. Whether you’re a beginner or an experienced developer, this guide will walk you through the essential steps to leverage C# for building intelligent systems. We will explore everything from understanding the C# ecosystem and data pre-processing to deploying AI models and real-world applications.
Understanding the C# Ecosystem for AI
.NET Framework and .NET Core
The first step in AI development with C# is understanding the platforms it runs on:
- .NET Framework: This is the traditional platform for C# development, primarily for building Windows applications. It offers extensive libraries and support for desktop and web applications but is limited to Windows environments, making it less flexible for AI projects that may require cross-platform deployment.
- .NET Core: A more modern and versatile platform,.NET Core is open-source and supports cross-platform development, running on Windows, Linux, and macOS. For AI applications,.NET Core’s performance and scalability make it the preferred choice, especially when deploying models in cloud environments or microservice architectures.
ML.NET
ML.NET is Microsoft’s machine learning framework designed for .NET developers. It allows you to create, train, and deploy machine learning models using C#. Key features include:
- AutoML: This feature automates the process of selecting the best machine learning model and hyperparameters for your data, making it easier for developers with less experience in data science.
- Model Builder: A GUI tool that simplifies model training and deployment without requiring extensive coding knowledge. It’s particularly useful for quickly prototyping and testing ideas.
- Seamless Integration: ML.NET integrates well with other .NET libraries, enabling you to incorporate machine learning models into your existing C# applications with minimal friction.
Third-party Libraries
While ML.NET is powerful, integrating third-party libraries can extend the capabilities of C# in AI development:
- TensorFlow.NET: This library brings the power of TensorFlow, one of the most popular deep learning frameworks, to the .NET ecosystem. With TensorFlow.NET, you can build and train complex neural networks in C# without needing to switch to Python.
- Accord.NET: A comprehensive framework for scientific computing, Accord.NET includes machine learning, statistical analysis, and signal processing. It’s ideal for developing AI applications that require advanced mathematical functions or real-time processing.
- Math.NET Numerics: This library provides advanced mathematical functions such as linear algebra, probability, and statistics, which are essential for implementing custom AI algorithms or performing complex data transformations.
Building the Foundation: Data Preprocessing
Data Acquisition
The quality and relevance of your data are crucial for the success of AI models. Data acquisition involves gathering data from various sources, such as databases, APIs, web scraping, and publicly available datasets. It’s important to ensure that the data you collect is relevant to the problem you’re trying to solve and is available in sufficient quantity to train effective models.
Data Cleaning
Data cleaning is a critical step that involves dealing with missing values, outliers, and inconsistencies in the dataset. Techniques include:
- Imputation: Filling in missing values using methods like mean, median, or mode imputation.
- Outlier Detection: Identifying and possibly removing or adjusting data points that deviate significantly from other observations, as these can skew the results of your model.
- Normalization and Standardization: Scaling features to a consistent range, which is particularly important for algorithms that are sensitive to the magnitude of data, such as neural networks.
Feature Engineering
Feature engineering involves transforming raw data into features that better represent the underlying problem to the model. This step can include:
- Scaling and Normalization: Adjusting the range of numerical features to ensure consistent scales across all features, which is crucial for algorithms like SVMs and neural networks.
- Encoding Categorical Variables: Converting categorical data into numerical values using techniques like one-hot encoding or label encoding, making it possible for algorithms to process this information.
- Feature Creation: Combining existing features to create new ones that can capture complex relationships within the data, thereby improving model performance.
Data Splitting
To evaluate your AI models properly, you should split your data into distinct sets:
- Training Set: Used to train the model and learn the patterns from the data.
- Validation Set: Used to tune model hyperparameters and prevent overfitting by providing feedback during the training process.
- Testing Set: Used for final evaluation, this set ensures that the model’s performance is assessed on unseen data, giving a realistic estimate of its generalization ability.
Core Machine Learning Algorithms with C#
Linear Regression
Linear regression is one of the simplest yet powerful algorithms used for predicting continuous outcomes. It models the relationship between a dependent variable and one or more independent variables:
- Simple linear regression: involves a single predictor variable. For example, predicting house prices based on size.
- Multiple Linear Regression: extends the model to include multiple predictor variables, such as predicting house prices based on size, location, and age.
Logistic Regression
Logistic regression is used for binary classification problems where the outcome is categorical (e.g., yes/no, true/false). It’s a foundational algorithm for tasks like spam detection or disease diagnosis. This section would cover:
- Binary Classification: Building a logistic regression model to classify data into one of two categories.
- Multiclass Classification: Extending logistic regression to handle multiple classes using techniques like one-vs-all or softmax regression.
Decision Trees and Random Forests
Decision trees are intuitive models that split the data into branches based on feature values. Random forests, an ensemble of decision trees, offer improved accuracy by combining the results of multiple trees:
- Decision Tree Construction: Implementing decision trees to make predictions by recursively splitting the data based on the most significant features.
- Random Forests: Combining the outputs of multiple decision trees to form a more robust model that reduces overfitting and improves accuracy.
Support Vector Machines (SVMs)
SVMs are powerful for both classification and regression tasks, particularly when the data has clear margins of separation. This section would explain:
- SVM Theory: Understanding the mathematical foundation behind SVMs, including the concept of hyperplanes and support vectors.
- Implementing SVMs in C#: Using libraries like Accord.NET to build and train SVM models for tasks such as image classification or spam detection.
Neural Networks
Neural networks are the foundation of deep learning, capable of solving complex problems by mimicking the human brain’s structure. This section would introduce:
- Neural Network Architecture: Understanding the layers, neurons, and activation functions that make up a neural network.
- Building Neural Networks in C#: Implementing neural networks using libraries like TensorFlow.NET or Accord.NET, with examples of simple tasks like digit recognition or sentiment analysis.
Deep Learning with C#
TensorFlow.NET
TensorFlow.NET is a .NET binding for TensorFlow, hiring C# developers to create and train deep learning models.
- Getting Started with TensorFlow.NET: Installing the library, setting up the environment, and understanding the basic concepts of TensorFlow.
- Building Deep Learning Models: Examples of creating and training models such as feedforward neural networks, convolutional neural networks (CNNs), and recurrent neural networks (RNNs).
Keras.NET
Keras.NET provides a high-level API to build neural networks more easily, offering a simplified syntax compared to TensorFlow:
- Using Keras.NET: Step-by-step guidance on building and training models, making it accessible for those who prefer a more straightforward approach to deep learning.
- Transfer Learning with Keras.NET: Applying pre-trained models to new tasks, reducing the need for extensive data and computation.
Convolutional Neural Networks (CNNs)
CNNs are specialized neural networks designed to process structured grid data, such as images:
- CNN Architecture: Understanding the different layers that make up a CNN, including convolutional layers, pooling layers, and fully connected layers.
- Implementing CNNs in C#: Building image recognition models using TensorFlow.NET or Keras.NET, with examples like classifying handwritten digits or detecting objects in images.
Recurrent Neural Networks (RNNs)
RNNs are designed to handle sequential data, making them ideal for tasks like time series forecasting and natural language processing (NLP):
- RNN Structure: Understanding how RNNs maintain information over time through loops in the network.
- Implementing RNNs in C#: Building models for tasks such as sentiment analysis, language modeling, or stock price prediction using libraries like TensorFlow.NET.
Deploying AI Models
Model Optimization
Before deploying your AI models, it’s essential to optimize them for performance:
- Pruning: Removing unnecessary parameters and nodes to reduce the model’s size without significantly impacting accuracy.
- Quantization: Reducing the precision of the model’s weights and biases, which can significantly decrease memory usage and increase inference speed, particularly on edge devices.
- Hyperparameter Tuning: Experimenting with different hyperparameters to find the best configuration for your model, ensuring it performs optimally.
Model Deployment
Deploying AI models involves making them accessible to users or other systems:
- Web Services: Using ASP.NET Core to deploy models as RESTful APIs, allowing them to be consumed by web applications or other services.
- Desktop Applications: Integrating models into desktop software using WPF or WinForms, providing AI-driven features directly on the user’s machine.
- Edge Deployment: Deploying models on edge devices such as IoT devices, ensuring low latency and reducing the need for constant internet connectivity.
Monitoring and Maintenance
Once deployed, AI models require continuous monitoring and maintenance:
- Performance Monitoring: Tracking metrics like accuracy, response time, and resource usage to ensure the model continues to meet performance expectations.
- Model Retraining: Periodically updating the model with new data to adapt to changing conditions or improve accuracy.
- Versioning: Keeping track of different versions of the model to manage updates, rollbacks, and ensure reproducibility.
Conclusion
Mastering AI development with C# opens up a world of possibilities for creating intelligent systems that can transform industries. By understanding the C# ecosystem, mastering core algorithms, and learning how to deploy and maintain models, you can build cutting-edge AI applications. Whether you’re looking to implement AI in finance, healthcare, manufacturing, or any other field, C# provides the tools and frameworks needed to turn your ideas into reality.